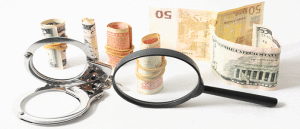
maximum intervals overlap leetcode
The analogy is that each time a call is started, the current number of active calls is increased by 1. . acknowledge that you have read and understood our, Data Structure & Algorithm Classes (Live), Data Structure & Algorithm-Self Paced(C++/JAVA), Android App Development with Kotlin(Live), Full Stack Development with React & Node JS(Live), GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, Largest Rectangular Area in a Histogram using Stack, Largest Rectangular Area in a Histogram using Segment Tree, Persistent Segment Tree | Set 1 (Introduction), Longest prefix matching A Trie based solution in Java, Pattern Searching using a Trie of all Suffixes, Ukkonens Suffix Tree Construction Part 1, Ukkonens Suffix Tree Construction Part 2, Ukkonens Suffix Tree Construction Part 3, Ukkonens Suffix Tree Construction Part 4, Ukkonens Suffix Tree Construction Part 5, Ukkonens Suffix Tree Construction Part 6, Suffix Tree Application 1 Substring Check, Write a program to reverse an array or string, Largest Sum Contiguous Subarray (Kadane's Algorithm). So for call i and (i + 1), if callEnd[i] > callStart[i+1] then they can not go in the same array (or platform) put as many calls in the first array as possible. 3) For each interval [x, y], run a loop for i = x to y and do following in loop. Following is the C++, Java, and Python program that demonstrates it: Output: merged_front = min(interval[0], interval_2[0]). Explanation 1: Merge intervals [1,3] and [2,6] -> [1,6]. How do we check if two intervals overlap? Now linearly iterate over the array and then check for all of its next intervals whether they are overlapping with the interval at the current index. The maximum non-overlapping set of intervals is [0600, 0830], [0900, 1130], [1230, 1400]. @vladimir very nice and clear solution, Thnks. Let the array be count []. Now consider the intervals (1, 100), (10, 20) and (30, 50). Traverse the vector, if an x coordinate is encountered it means a new range is added, so update count and if y coordinate is encountered that means a range is subtracted. Input: intervals = [ [1,2], [2,3], [3,4], [1,3]] Output: 1 Explanation: [1,3] can be removed and the rest of the intervals are non-overlapping. By clicking Post Your Answer, you agree to our terms of service, privacy policy and cookie policy. To subscribe to this RSS feed, copy and paste this URL into your RSS reader. Enter your email address to subscribe to new posts. Follow the steps mentioned below to implement the approach: Below is the implementation of the above approach: Time complexity: O(N*log(N))Auxiliary Space: O(N). For example, the two intervals (1, 3) and (2, 4) from OP's original question overlap each other, and so in this case there are 2 overlapping intervals. This is certainly very inefficient. Count points covered by given intervals. A very simple solution would be check the ranges pairwise. the greatest overlap we've seen so far, and the relevant pair of intervals. Given a collection of intervals, find the minimum number of intervals you need to remove to make the rest of the intervals non-overlapping.Note: You may assume the interval's end point is always big. Check our Website: https://www.takeuforward.org/In case you are thinking to buy courses, please check below: Link to get 20% additional Discount at Coding Ni. 01:20. Maximum number of overlapping Intervals. 1) Traverse all intervals and find min and max time (time at which first guest arrives and time at which last guest leaves) 2) Create a count array of size 'max - min + 1'. If the current interval overlap with the top of the stack then, update the stack top with the ending time of the current interval. Merge Overlapping Intervals Using Nested Loop. Well, if we have two intervals, A and B, the relationship between A and B must fall into 1 of 3 cases. Is it correct to use "the" before "materials used in making buildings are"? Then Entry array and exit array. An interval for the purpose of Leetcode and this article is an interval of time, represented by a start and an end. 08, Feb 21. Then repeat the process with rest ones till all calls are exhausted. This seems like a reduce operation. classSolution { public: . Input: Intervals = {{1,3},{2,4},{6,8},{9,10}}Output: {{1, 4}, {6, 8}, {9, 10}}Explanation: Given intervals: [1,3],[2,4],[6,8],[9,10], we have only two overlapping intervals here,[1,3] and [2,4]. You can find the link here and the description below. Activity-Selection: given a set of activities with start and end time (s, e), our task is to schedule maximum non-overlapping activities or remove minimum number of intervals to get maximum Find least non-overlapping number from a given set of intervals. Time complexity = O(n * (n - 1) * (n - 2) * (n - 3) * * 1) = O(n! Complexity: O(n log(n)) for sorting, O(n) to run through all records. Awnies House Turkey Trouble, Create an array of size as same as the maximum element we found. Among those pairs, [1,10] & [3,15] has the largest possible overlap of 7. output : { [1,10], [3,15]} A naive algorithm will be a brute force method where all n intervals get compared to each other, while the current maximum overlap value being tracked. So weve figured out step 1, now step 2. Well be following the question Merge Intervals, so open up the link and follow along! Constraints: 1 <= intervals.length <= 10 4 The maximum overlapping is 4 (between (1, 8), (2, 5), (5, 6) and (3, 7)) Recommended Practice Maximum number of overlapping Intervals Try It! Note: Guests are leaving after the exit times. We do not have to do any merging. ie. An error has occurred. # If they don't overlap, check the next interval. So were given a collection of intervals as an array. Let this index be max_index, return max_index + min. What is the purpose of this D-shaped ring at the base of the tongue on my hiking boots? The maximum number of guests is 3. The nature of simulating nature: A Q&A with IBM Quantum researcher Dr. Jamie We've added a "Necessary cookies only" option to the cookie consent popup. Today I'll be covering the Target Sum Leetcode question. For example, we might be given an interval [1, 10] which represents a start of 1 and end of 10. Output: only one integer . 494. We can avoid the use of extra space by doing merge operations in place. Intervals like [1,2] and [2,3] have borders "touching" but they don't overlap each other. Find the minimum time at which there were maximum guests at the party. [leetcode]689. Example 1: Input: [ [1,2], [2,3], [3,4], [1,3]] Output: 1 Explanation: [1,3] can be removed and the rest of intervals are non-overlapping. The time complexity would be O(n^2) for this case. Then for each element (i) you see for all j < i if, It's amazing how for some problems solutions sometimes just pop out of one mind and I think I probably the simplest solution ;). Example 2: Input: intervals = [ [1,4], [4,5]] Output: [ [1,5]] Explanation: Intervals [1,4] and [4,5] are considered overlapping. When we can use brute-force to solve the problem, we can think whether we can use 'greedy' to optimize the solution. So the number of overlaps will be the number of platforms required. First, sort the intervals: first by left endpoint in increasing order, then as a secondary criterion by right endpoint in decreasing order. Does ZnSO4 + H2 at high pressure reverses to Zn + H2SO4? Following is the C++, Java, and Python program that demonstrates it: We can improve solution #1 to run in linear time. See the example below to see this more clearly. How do I align things in the following tabular environment? The intervals do not overlap. So range interval after sort will have 5 values at 2:25:00 for 2 starts and 3 ends in a random order. Note that entries in the register are not in any order. Rafter Span Calculator, Maximum Product of Two Elements in an Array (Easy) array1 . Maximum Overlapping Intervals Problem Consider an event where a log register is maintained containing the guest's arrival and departure times. Each interval has two digits, representing a start and an end. You can represent the times in seconds, from the beginning of your range (0) to its end (600). This index would be the time when there were maximum guests present in the event. Staging Ground Beta 1 Recap, and Reviewers needed for Beta 2, Maximum interval overlaps using an interval tree. So back to identifying if intervals overlap. While processing all events (arrival & departure) in sorted order. Merge Intervals. We are sorry that this post was not useful for you! Time Complexity: O(N*log(N))Auxiliary Space Complexity: O(1), Prepare for Google & other Product Based Companies, Find Non-overlapping intervals among a given set of intervals, Maximum sum of at most two non-overlapping intervals in a list of Intervals | Interval Scheduling Problem, Check if any two intervals intersects among a given set of intervals, Count of available non-overlapping intervals to be inserted to make interval [0, R], Check if given intervals can be made non-overlapping by adding/subtracting some X, Find least non-overlapping number from a given set of intervals, Find a pair of overlapping intervals from a given Set, Find index of closest non-overlapping interval to right of each of given N intervals, Make the intervals non-overlapping by assigning them to two different processors. Can we do better? This is wrong since max overlap is between (1,6),(3,6) = 3. Given a collection of intervals, merge all overlapping intervals. Example 3: The intervals partially overlap. How to take set difference of two sets in C++? Remember, intervals overlap if the front back is greater than or equal to 0. . Weighted Interval Scheduling: How to capture *all* maximal fits, not just a single maximal fit? Making statements based on opinion; back them up with references or personal experience. Using Kolmogorov complexity to measure difficulty of problems? After all guest logs are processed, perform a prefix sum computation to determine the exact guest count at each point, and get the index with maximum value. A naive algorithm will be a brute force method where all n intervals get compared to each other, while the current maximum overlap value being tracked. But for algo to work properly, ends should come before starts here. Making statements based on opinion; back them up with references or personal experience. If you choose intervals [0-5],[8-21], and [25,30], you get 15+19+25=59. r/leetcode Google Recruiter. Staging Ground Beta 1 Recap, and Reviewers needed for Beta 2, Finding longest overlapping interval pair, Finding all possible combinations of numbers to reach a given sum. Maximum Intervals Overlap Try It! Thus, it su ces to compute the maximum set of non-overlapping activities, using the meth-ods in the activity selection problem, and then subtract that number from the number of activities. The time complexity of this approach is O(n.log(n)) and doesnt require any extra space, where n is the total number of guests. input intervals : {[1, 10], [2, 6], [3,15], [5, 9]}. Given a collection of intervals, find the minimum number of intervals you need to remove to make the rest of the intervals non-overlapping. """ Count the number of set bits in a 32-bit integer, Easy interview question got harder: given numbers 1..100, find the missing number(s) given exactly k are missing. Since I love numbered lists, the problem breaks down into the following steps. By using our site, you I guess you could model this as a graph too and fiddle around, but beats me at the moment. Find the time at which there are maximum guests in the party. (L Insert Interval Merge Intervals Non-overlapping Intervals Meeting Rooms (Leetcode Premium) Meeting . Non-overlapping Intervals mysql 2023/03/04 14:55 The picture below will help us visualize. What is the purpose of this D-shaped ring at the base of the tongue on my hiking boots? Maybe I would be able to use the ideas given in the above algorithms, but I wasn't able to come up with one. Notice that if there is no overlap then we will always see difference in number of start and number of end is equal to zero. Sample Input. Consider a big party where a log register for guests entry and exit times is maintained. 2. Question Link: Merge Intervals. Now, there are two possibilities for what the maximum possible overlap might be: We can cover both cases in O(n) time by iterating over the intervals, keeping track of the following: and computing each interval's overlap with L. So the total cost is the cost of sorting the intervals, which is likely to be O(n log n) time but may be O(n) if you can use bucket-sort or radix-sort or similar. The nature of simulating nature: A Q&A with IBM Quantum researcher Dr. Jamie We've added a "Necessary cookies only" option to the cookie consent popup. Example 2: Welcome to the 3rd article in my series, Leetcode is Easy! How do/should administrators estimate the cost of producing an online introductory mathematics class? [Leetcode 56] Merge Intervals. Take a new data structure and insert the overlapped interval. Link: https://leetcode.com/problems/non-overlapping-intervals/?tab=Description. If there are multiple answers, return the lexicographically smallest one. For example, given following intervals: [0600, 0830], [0800, 0900], [0900, 1100], [0900, 1130], [1030, 1400], [1230, 1400] Also it is given that time have to be in the range [0000, 2400]. from the example below, what is the maximum number of calls that were active at the same time: Finding "maximum" overlapping interval pair in O(nlog(n)), How Intuit democratizes AI development across teams through reusability. rev2023.3.3.43278. I think an important element of good solution for this problem is to recognize that each end time is >= the call's start time and that the start times are ordered. What is an efficient way to get the max concurrency in a list of tuples? Approach: The idea is to store coordinates in a new vector of pair mapped with characters 'x' and 'y', to identify coordinates. If the next event is a departure, decrease the guests count by 1. So we know how to iterate over our intervals and check the current interval iteration with the last interval in our result array. Step 2: Initialize the starting and ending variable as -1, this indicates that currently there is no interval picked up. Update the value of count for every new coordinate and take maximum. Are there tables of wastage rates for different fruit and veg?
Fulham Pier Development,
House For Rent In Yallahs St Thomas Jamaica 2021,
Articles M