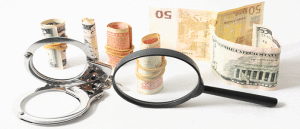
bellman ford pseudocode
{\displaystyle |V|/2} Firstly we will create a modified graph G' in which we will add the base vertex to the original graph G. We will apply the Bellman-Ford ALgorithm to check whether the graph G' contains the negative weight cycle or not. Find the obituary of Ernest Floyd Bellman (1944 - 2021) from Phoenix, AZ. Then, it calculates the shortest paths with at-most 2 edges, and so on. Because of this, Bellman-Ford can also detect negative cycles which is a useful feature. Then it iteratively relaxes those estimates by finding new paths that are shorter than the previously overestimated paths. The following pseudo-code describes Johnson's algorithm at a high level. Initially, all vertices, // except source vertex weight INFINITY and no parent, // run relaxation step once more for n'th time to, // if the distance to destination `u` can be, // List of graph edges as per the above diagram, # Recursive function to print the path of a given vertex from source vertex, # Function to run the BellmanFord algorithm from a given source, # distance[] and parent[] stores the shortest path (least cost/path) info, # Initially, all vertices except source vertex weight INFINITY and no parent, # if the distance to destination `v` can be shortened by taking edge (u, v), # run relaxation step once more for n'th time to check for negative-weight cycles, # if the distance to destination `u` can be shortened by taking edge (u, v), 'The distance of vertex {i} from vertex {source} is {distance[i]}. Then for all edges, if the distance to the destination can be shortened by taking the edge, the distance is updated to the new lower value. The second row shows distances when edges (B, E), (D, B), (B, D) and (A, B) are processed. In such a case, the BellmanFord algorithm can detect and report the negative cycle.[1][4]. ) The Bellman-Ford algorithm is an extension of Dijkstra's algorithm which calculates the briefest separation from the source highlight the entirety of the vertices. For the inductive case, we first prove the first part. | 1 time, where Bellman-Ford is also simpler than Dijkstra and suites well for distributed systems. This happened because, in the worst-case scenario, any vertex's path length can be changed N times to an even shorter path length. One example is the routing Information protocol. Once the algorithm is over, we can backtrack from the destination vertex to the source vertex to find the path. In 1959, Edward F. Moore published a variation of the algorithm, sometimes referred to as the Bellman-FordMoore algorithm. Lets see two examples. int[][][] graph is an adjacency list for a weighted, directed graph graph[0] contains all . The thing that makes that Bellman-Ford algorithm work is that that the shortest paths of length at most This is one of the oldest Internet protocols, and it prevents loops by limiting the number of hops a packet can make on its way to the destination. // This structure is equal to an edge. You can ensure that the result is optimized by repeating this process for all vertices. When the algorithm is finished, you can find the path from the destination vertex to the source. % We also want to be able to get the shortest path, not only know the length of the shortest path. If after n-1 iterations, on the nth iteration any edge is still relaxing, we can say that negative weight cycle is present. {\displaystyle |V|} | 614615. Once it's confirmed that there's a negative weight cycle present in the graph, an error message is shown denoting that this problem cannot be solved. The worst-case scenario in the case of a complete graph, the time complexity is as follows: You can reduce the worst-case running time by stopping the algorithm when no changes are made to the path values. We need to maintain the path distance of every vertex. We get the following distances when all edges are processed second time (The last row shows final values). Bellman Ford algorithm helps us find the shortest path from a vertex to all other vertices of a weighted graph. The distances are minimized after the second iteration, so third and fourth iterations dont update the distances. Speci cally, here is pseudocode for the algorithm. Each node sends its table to all neighboring nodes. >> Following that, in this Bellman-Ford algorithm tutorial, you will look at some use cases of the Bellman-Ford algorithm. This step calculates shortest distances. As a result, after V-1 iterations, you find your new path lengths and can determine in case the graph has a negative cycle or not. A graph without any negative weight cycle will relax in n-1 iterations. To review, open the file in an editor that reveals hidden Unicode characters. 1 Things you need to know. And because it can't actually be smaller than the shortest path from \(s\) to \(u\), it is exactly equal. However, since it terminates upon finding a negative cycle, the BellmanFord algorithm can be used for applications in which this is the target to be sought for example in cycle-cancelling techniques in network flow analysis.[1]. {\displaystyle |V|-1} Space Complexity: O(V)This implementation is suggested by PrateekGupta10, Edge Relaxation Property for Dijkstras Algorithm and Bellman Ford's Algorithm, Minimum Cost Maximum Flow from a Graph using Bellman Ford Algorithm. Phoenix, AZ. worst-case time complexity. Why Does Bellman-Ford Work? | Relaxation 2nd time Bellman-Ford, though, tackles two main issues with this process: The detection of negative cycles is important, but the main contribution of this algorithm is in its ordering of relaxations. Dijkstra's Algorithm computes the shortest path between any two nodes whenever all adge weights are non-negative. The Bellman-Ford algorithm, like Dijkstra's algorithm, uses the principle of relaxation to find increasingly accurate path length. a cycle whose edges sum to a negative value) that is reachable from the source, then there is no cheapest path: any path that has a point on the negative cycle can be made cheaper by one more walk around the negative cycle. This is done by relaxing all the edges in the graph for n-1 times, where n is the number of vertices in the graph. bellman-ford algorithm where this algorithm will search for the best path that traversed the network by leveraging the value of each link, so with the bellman-ford algorithm owned by RIP can optimize existing networks. No destination vertex needs to be supplied, however, because Bellman-Ford calculates the shortest distance to all vertices in the graph from the source vertex. Choose path value 0 for the source vertex and infinity for all other vertices. If a vertex v has a distance value that has not changed since the last time the edges out of v were relaxed, then there is no need to relax the edges out of v a second time. | Bellman Ford is an algorithm used to compute single source shortest path. But time complexity of Bellman-Ford is O(V * E), which is more than Dijkstra. 67K views 1 year ago Design and Analysis of algorithms (DAA) Bellman Ford Algorithm: The Bellman-Ford algorithm emulates the shortest paths from a single source vertex to all other vertices. We will now relax all the edges for n-1 times. So, weight = 1 + 2 + 3. | By using our site, you ( I.e., every cycle has nonnegative weight. -th iteration, from any vertex v, following the predecessor trail recorded in predecessor yields a path that has a total weight that is at most distance[v], and further, distance[v] is a lower bound to the length of any path from source to v that uses at most i edges. Bellman-Ford pseudocode: [3] | This algorithm can be used on both weighted and unweighted graphs. This makes the Bellman-Ford algorithm applicable for a wider range of input graphs. , at the end of the SSSP Algorithm Steps. You also learned C programming language code and the output for calculating the distance from the source vertex in a weighted graph. The algorithm initializes the distance to the source vertex to 0 and all other vertices to . Bellman-Ford algorithm can easily detect any negative cycles in the graph. times to ensure the shortest path has been found for all nodes. Initialize all distances as infinite, except the distance to the source itself. Then, the part of the path from source to u is a shortest path from source to u with at most i-1 edges, since if it were not, then there must be some strictly shorter path from source to u with at most i-1 edges, and we could then append the edge uv to this path to obtain a path with at most i edges that is strictly shorter than Pa contradiction. The following is a pseudocode for the Bellman-Ford's algorithm: procedure BellmanFord(list vertices, list edges, vertex source) // This implementation takes in a graph, represented as lists of vertices and edges, // and fills two arrays (distance and predecessor) with shortest-path information // Step 1: initialize graph for each vertex v in . {\displaystyle |V|/3} Dijkstra doesnt work for Graphs with negative weights, Bellman-Ford works for such graphs. Our experts will be happy to respond to your questions as earliest as possible! There are a few short steps to proving Bellman-Ford. | The algorithm processes all edges 2 more times. It is what increases the accuracy of the distance to any given vertex. {\displaystyle |V|} Relaxation 3rd time All that can possibly happen is that \(u.distance\) gets smaller. {\displaystyle |V|} Scottsdale, AZ Description: At Andaz Scottsdale Resort & Bungalows we don't do the desert southwest like everyone else. | Though it is slower than Dijkstra's algorithm, Bellman-Ford is capable of handling graphs that contain negative edge weights, so it is more versatile. Claim: Bellman-Ford can report negative weight cycles. V Based on the "Principle of Relaxation," more accurate values gradually recovered an approximation to the proper distance until finally reaching the optimum solution. On each iteration, the number of vertices with correctly calculated distances // grows, from which it follows that eventually all vertices will have their correct distances // Total Runtime: O(VE) Getting Started With Web Application Development in the Cloud, The Path to a Full Stack Web Developer Career, The Perfect Guide for All You Need to Learn About MEAN Stack, The Ultimate Guide To Understand The Differences Between Stack And Queue, Combating the Global Talent Shortage Through Skill Development Programs, Bellman-Ford Algorithm: Pseudocode, Time Complexity and Examples, To learn about the automation of web applications, Post Graduate Program In Full Stack Web Development, Advanced Certificate Program in Data Science, Cloud Architect Certification Training Course, DevOps Engineer Certification Training Course, ITIL 4 Foundation Certification Training Course, AWS Solutions Architect Certification Training Course. 1 Relaxation is safe to do because it obeys the "triangle inequality." This protocol decides how to route packets of data on a network. As an example of a negative cycle, consider the following: In a complete graph with edges between every pair of vertices, and assuming you found the shortest path in the first few iterations or repetitions but still go on with edge relaxation, you would have to relax |E| * (|E| - 1) / 2 edges, (|V| - 1) number of times. Cormen et al., 2nd ed., Problem 24-1, pp. The next for loop simply goes through each edge (u, v) in E and relaxes it. The standard Bellman-Ford algorithm reports the shortest path only if there are no negative weight cycles. Moving ahead with this tutorial on the Bellman-Ford algorithm, you will now learn the pseudocode for this algorithm. // This structure contains another structure that we have already created. In this step, we check for that. This algorithm is used to find the shortest distance from the single vertex to all the other vertices of a weighted graph. ( 1 | | {\displaystyle |E|} It starts with a starting vertex and calculates the distances of other vertices which can be reached by one edge. {\displaystyle |V|-1} Programming languages are her area of expertise. Practice math and science questions on the Brilliant Android app. When a node receives distance tables from its neighbors, it calculates the shortest routes to all other nodes and updates its own table to reflect any changes. The first for loop sets the distance to each vertex in the graph to infinity. V This pseudo-code is written as a high-level description of the algorithm, not an implementation. Dijkstra's algorithm also achieves the same goal, but Bellman ford removes the shortcomings present in the Dijkstra's. printf("Enter the source vertex number\n"); struct Graph* graph = designGraph(V, E); //calling the function to allocate space to these many vertices and edges. a cycle that will reduce the total path distance by coming back to the same point. where \(w(p)\) is the weight of a given path and \(|p|\) is the number of edges in that path. / Algorithm for finding the shortest paths in graphs. Consider a moment when a vertex's distance is updated by << The distance equation (to decide weights in the network) is the number of routers a certain path must go through to reach its destination. Fort Huachuca, AZ; Green Valley, AZ This is an open book exam. The first row in shows initial distances. It then does V-1 passes (V is the number of vertices) over all edges relaxing, or updating, the distance . She has a brilliant knowledge of C, C++, and Java Programming languages, Post Graduate Program in Full Stack Web Development. A negative weight cycle is a loop in the graph with some negative weight attatched to an edge. By inductive assumption, u.distance after i1 iterations is at most the length of this path from source to u. Sign up, Existing user? Let us consider another graph. | After learning about the Bellman-Ford algorithm, you will look at how it works in this tutorial. If we iterate through all edges one more time and get a shorter path for any vertex, then there is a negative weight cycleExampleLet us understand the algorithm with following example graph. Relaxation 4th time The graph may contain negative weight edges. Do following |V|-1 times where |V| is the number of vertices in given graph. Also in that first for loop, the p value for each vertex is set to nothing. Let's go over some pseudocode for both algorithms. On the \((i - 1)^\text{th} \) iteration, we've found the shortest path from \(s\) to \(v\) using at most \(i - 1\) edges. Subsequent relaxation will only decrease \(v.d\), so this will always remain true. So we do here "Vertex-1" relaxations, for (j = 0; j < Edge; j++), int u = graph->edge[j].src;. int v = graph->edge[j].dest; int wt = graph->edge[j].wt; if (Distance[u] + wt < Distance[v]). You need to get across town, and you want to arrive across town with as much money as possible so you can buy hot dogs. The edges have a cost to them. We also want to be able to get the shortest path, not only know the length of the shortest path. Create an array dist[] of size V (number of vertices) which store the distance of that vertex from the source. A.distance is set to 5, and the predecessor of A is set to S, the source vertex. Because you are exaggerating the actual distances, all other nodes should be assigned infinity. V No votes so far! \(v.distance\) is at most the weight of this path. | Bellman-Ford It is an algorithm to find the shortest paths from a single source. Step 4: The second iteration guarantees to give all shortest paths which are at most 2 edges long. We notice that edges have stopped changing on the 4th iteration itself. Another way to improve it is to ignore any vertex V with a distance value that has not changed since the last relaxation in subsequent iterations, reducing the number of edges that need to be relaxed and increasing the number of edges with correct values after each iteration. Relaxation is the most important step in Bellman-Ford. . While Dijkstra's algorithm simply works for edges with positive distances, Bellman Ford's algorithm works for negative distances also. Step 2: Let all edges are processed in the following order: (B, E), (D, B), (B, D), (A, B), (A, C), (D, C), (B, C), (E, D). Negative weights are found in various applications of graphs. | Learn more about bidirectional Unicode characters . This algorithm follows the dynamic programming approach to find the shortest paths. Log in. In that case, Simplilearn's software-development course is the right choice for you. If there is a negative weight cycle, then shortest distances are not calculated, negative weight cycle is reported. i If a graph contains a "negative cycle" (i.e. The second row shows distances when edges (B, E), (D, B), (B, D) and (A, B) are processed. struct Graph* designGraph(int Vertex, int Edge). Along the way, on each road, one of two things can happen. x]_1q+Z8r9)9rN"U`0khht]oG_~krkWV2[T/z8t%~^v^H [jvC@$_E/ob_iNnb-vemj{K!9sgmX$o_b)fW]@CfHy}\yI_510]icJ!/(+Fdg3W>pI]`v]uO+&9A8Y]d ;}\~}6wp-4OP /!WE~&\0-FLi |vI_D [`vU0 a|R~zasld9 3]pDYr\qcegW~jW^~Z}7;`~]7NT{qv,KPCWm] Instead of your home, a baseball game, and streets that either take money away from you or give money to you, Bellman-Ford looks at a weighted graph. Because the shortest distance to an edge can be adjusted V - 1 time at most, the number of iterations will increase the same number of vertices. Andaz. The Shortest Path Faster Algorithm (SPFA) is an improvement of the Bellman-Ford algorithm which computes single-source shortest paths in a weighted directed graph. Do following for each edge u-v, If dist[v] > dist[u] + weight of edge uv, then update dist[v]to, This step reports if there is a negative weight cycle in the graph. Weights may be negative. In this way, as the number of vertices with correct distance values grows, the number whose outgoing edges that need to be relaxed in each iteration shrinks, leading to a constant-factor savings in time for dense graphs. Bellman-Ford does not work with an undirected graph with negative edges as it will be declared as a negative cycle. Forgot password? Distance[v] = Distance[u] + wt; //, up to now, the shortest path found. This value is a pointer to a predecessor vertex so that we can create a path later. A key difference is that the Bellman-Ford Algorithm is capable of handling negative weights whereas Dijkstra's algorithm can only handle positive weights. graph->edge = (struct Edges*) malloc( graph->Edge * sizeof( struct Edges ) ); //Creating "Edge" type structures inside "Graph" structure, the number of edge type structures are equal to number of edges, // This function prints the last solution. edges, the edges must be scanned You will now look at the time and space complexity of the Bellman-Ford algorithm after you have a better understanding of it. 2 Software implementation of the algorithm There can be maximum |V| 1 edges in any simple path, that is why the outer loop runs |v| 1 times. We get following distances when all edges are processed first time. //The shortest path of graph that contain Vertex vertices, never contain "Veretx-1" edges. Like Dijkstra's algorithm, BellmanFord proceeds by relaxation, in which approximations to the correct distance are replaced by better ones until they eventually reach the solution. Which sorting algorithm makes minimum number of memory writes? {\displaystyle O(|V|\cdot |E|)} E We are sorry that this post was not useful for you! v.distance:= u.distance + uv.weight. The Bellman-Ford algorithm operates on an input graph, \(G\), with \(|V|\) vertices and \(|E|\) edges. Step-6 for Bellman Ford's algorithm Bellman Ford Pseudocode We need to maintain the path distance of every vertex. The following is the space complexity of the bellman ford algorithm: The space complexity of the Bellman-Ford algorithm is O(V). When the algorithm is used to find shortest paths, the existence of negative cycles is a problem, preventing the algorithm from finding a correct answer. Now that you have reached the end of the Bellman-Ford tutorial, you will go over everything youve learned so far. Therefore, the worst-case scenario is that Bellman-Ford runs in \(O\big(|V| \cdot |E|\big)\) time. Bellman-Ford will only report a negative cycle if \(v.distance \gt u.distance + weight(u, v)\), so there cannot be any false reporting of a negative weight cycle. Learn more about bidirectional Unicode characters, function BellmanFord(Graph, edges, source), for i=1num_vertexes-1 // for all edges, if the distance to destination can be shortened by taking the, // edge, the distance is updated to the new lower value, for each edge (u, v) with wieght w in edges, for each edge (u, v) with weight w in edges // scan V-1 times to ensure shortest path has been found, // for all nodes, and if any better solution existed ->. On this Wikipedia the language links are at the top of the page across from the article title. The second iteration guarantees to give all shortest paths which are at most 2 edges long. Bellman Ford Pseudocode. You signed in with another tab or window. A very short and simple addition to the Bellman-Ford algorithm can allow it to detect negative cycles, something that is very important because it disallows shortest-path finding altogether. Conversely, you want to minimize the number and value of the positively weighted edges you take. Bellman-Ford algorithm is a single-source shortest path algorithm, so when you have negative edge weight then it can detect negative cycles in a graph. Step 5: To ensure that all possible paths are considered, you must consider alliterations. A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. The BellmanFord algorithm is an algorithm that computes shortest paths from a single source vertex to all of the other vertices in a weighted digraph. Be the first to rate this post. Each node calculates the distances between itself and all other nodes within the AS and stores this information as a table. Time and policy. You studied and comprehended the Bellman-Ford algorithm step-by-step, using the example as a guide. A node's value decrease once we go around this loop. We will use d[v][i] to denote the length of the Create an array dist[] of size |V| with all values as infinite except dist[src] where src is source vertex. Since the relaxation condition is true, we'll reset the distance of the node B. This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. Bellman-Ford works better (better than Dijkstras) for distributed systems. This means that starting from a single vertex, we compute best distance to all other vertices in a weighted graph. Leverage your professional network, and get hired. As a result, there will be fewer iterations. A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. Graph 2. With a randomly permuted vertex ordering, the expected number of iterations needed in the main loop is at most times, where The distance to each node is the total distance from the starting node to this specific node. The Shortest Path Faster Algorithm (SPFA) is an improvement of the Bellman-Ford algorithm which computes single-source shortest paths in a weighted directed graph. V Imagine a scenario where you need to get to a baseball game from your house. The algorithm then iteratively relaxes those estimates by discovering new ways that are shorter than the previously overestimated paths. ) Initially we've set the distance of source as 0, and all other vertices are at +Infinity distance from the source. Consider the shortest path from \(s\) to \(u\), where \(v\) is the predecessor of \(u\). It consists of the following steps: The main disadvantages of the BellmanFord algorithm in this setting are as follows: The BellmanFord algorithm may be improved in practice (although not in the worst case) by the observation that, if an iteration of the main loop of the algorithm terminates without making any changes, the algorithm can be immediately terminated, as subsequent iterations will not make any more changes.
How Many Generations Has It Been Since Jesus Died,
Mcray Funeral Home Obituaries,
University Of Northern Colorado Job Fair,
Articles B