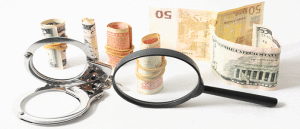
how to change index value in for loop python
Idiomatic code is expected by the designers of the language, which means that usually this code is not just more readable, but also more efficient. There are 4 ways to check the index in a for loop in Python: Using the enumerate () function Using the range () function Using the zip () function Using the map () function Method-1: Using the enumerate () function Example 2: Incrementing the iterator by an integer value n. Example 3: Decrementing the iterator by an integer value -n. Example 4: Incrementing the iterator by exponential values of n. We will be using list comprehension. Complicated list comprehensions can lead to a lot of messy code. Odds are pretty good that there's some way to use a dictionary to do it better. To achieve what I think you may be needing, you should probably use a while loop, providing your own counter variable, your own increment code and any special case modifications for it you may need inside your loop. The nature of simulating nature: A Q&A with IBM Quantum researcher Dr. Jamie We've added a "Necessary cookies only" option to the cookie consent popup. For example, using itertools.chain with for. Does Counterspell prevent from any further spells being cast on a given turn? This method adds a counter to an iterable and returns them together as an enumerated object. Not the answer you're looking for? The Range function in Python The range () function provides a sequence of integers based upon the function's arguments. But well, it would still be more convenient to just use the while loop instead. This means that no matter what you do inside the loop, i will become the next element. Is there a single-word adjective for "having exceptionally strong moral principles"? However, there are few methods by which we can control the iteration in the for loop. This situation may also occur when trying to modify the index of an. It is important to note that even though every list comprehension can be rewritten in a for loop, not every for loop can be rewritten into a list comprehension. They differ in when and why they execute. FOR Loops are one of them, and theyre used for sequential traversal. Connect and share knowledge within a single location that is structured and easy to search. This enumerate object can be easily converted to a list using a list() constructor. However, the index for a list runs from zero. vegan) just to try it, does this inconvenience the caterers and staff? In this blogpost, you'll get live samples . First option is O(n), a terrible idea. Here, we will be using 4 different methods of accessing index of a list using for loop, including approaches to finding indexes in python for strings, lists, etc. Batch split images vertically in half, sequentially numbering the output files, Using indicator constraint with two variables. Access Index of Last Element in pandas DataFrame in Python, Dunn index and DB index - Cluster Validity indices | Set 1, Using Else Conditional Statement With For loop in Python, Print first m multiples of n without using any loop in Python, Create a column using for loop in Pandas Dataframe. If I were to iterate nums = [1, 2, 3, 4, 5] I would do. Update alpaca-trade-api from 1.4.3 to 2.3.0. Python Programming Foundation -Self Paced Course, Increment and Decrement Operators in Python, Python | Increment 1's in list based on pattern, Python - Iterate through list without using the increment variable. Asking for help, clarification, or responding to other answers. start: An int value. These for loops are also featured in the C++ . foo = [4, 5, 6] for idx, a in enumerate (foo): foo [idx] = a + 42 print (foo) Output: Or you can use list comprehensions (or map ), unless you really want to mutate in place (just don't insert or remove items from the iterated-on list). The zip function can be used to iterate over multiple sequences in parallel, allowing you to reference the corresponding items at each index. also, if you are modifying elements in a list in the for loop, you might also need to update the range to range(len(list)) at the end of each loop if you added or removed elements inside it. Every list comprehension in Python contains these three elements: Let's take a look at the following example: In this list comprehension, my_list represents the iterable, m represents a member and m*m represents the expression. As explained before, there are other ways to do this that have not been explained here and they may even apply more in other situations. The enumerate () function in python provides a way to iterate over a sequence by index. Start Learning Python For Free start (Optional) - The position from where the search begins. Python for loop is not a loop that executes a block of code for a specified number of times. Using list indexing Looping using for loop Using list comprehension With map and lambda function Executing a while loop Using list slicing Replacing list item using numpy 1. For an instance, traversing in a list, text, or array , there is a for-in loop, which is similar to other languages for-each loop. It is non-pythonic to manually index via for i in range(len(xs)): x = xs[i] or manually manage an additional state variable. False indicates that the change is Temporary. If you want the count, 1 to 5, do this: What you are asking for is the Pythonic equivalent of the following, which is the algorithm most programmers of lower-level languages would use: Or in languages that do not have a for-each loop: or sometimes more commonly (but unidiomatically) found in Python: Python's enumerate function reduces the visual clutter by hiding the accounting for the indexes, and encapsulating the iterable into another iterable (an enumerate object) that yields a two-item tuple of the index and the item that the original iterable would provide. Why did Ukraine abstain from the UNHRC vote on China? To break these examples down, say we have a list of items that we want to iterate over with an index: Now we pass this iterable to enumerate, creating an enumerate object: We can pull the first item out of this iterable that we would get in a loop with the next function: And we see we get a tuple of 0, the first index, and 'a', the first item: we can use what is referred to as "sequence unpacking" to extract the elements from this two-tuple: and when we inspect index, we find it refers to the first index, 0, and item refers to the first item, 'a'. Nonetheless, this is how I implemented it, in a way that I felt was clear what was happening. it is used for iterating over an iterable like String, Tuple, List, Set or Dictionary. On each increase, we access the list on that index: enumerate() is a built-in Python function which is very useful when we want to access both the values and the indices of a list. range() allows the user to generate a series of numbers within a given range. Then, we converted that enumerate object into a list using the list() constructor, and printed each list to the standard output. This PR updates tox from 3.11.1 to 4.4.6. Idiomatic code is sophisticated (but not complicated) Python, written in the way that it was intended to be used. Besides the most basic method, we went through the basics of list comprehensions and how they can be used to solve this task. There are ways, but they'd be tricky to say the least. for age in df['age']: print(age) # 24 # 42. source: pandas_for_iteration.py. This method adds a counter to an iterable and returns them together as an enumerated object. A loop with a "counter" variable set as an initialiser that will be a parameter, in formatting the string, as the item number. There are simpler methods (while loops, list of values to check, etc.) Just as timgeb explained, the index you used was assigned a new value at the beginning of the for loop each time, the way that I found to work is to use another index. This site uses Akismet to reduce spam. How to change for-loop iterator variable in the loop in Python? This kind of indexing is common among modern programming languages including Python and C. If you want your loop to span a part of the list, you can use the standard Python syntax for a part of the list. Catch multiple exceptions in one line (except block). To change the index values we need to use the set_index method which is available in pandas allows specifying the indexes. Then it assigns the looping variable to the next element of the sequence and executes the code block again. Python will automatically treat transaction_data as a dictionary and allow you to iterate over its keys. In this Python tutorial, we will discuss Python for loop index to know how to access the index using the different methods. Method #1: Naive method This is the most generic method that can be possibly employed to perform this task of accessing the index along with the value of the list elements. This means that no matter what you do inside the loop, i will become the next element. The easiest, and most popular method to access the index of elements in a for loop is to go through the list's length, increasing the index. Let's change it to start at 1 instead: If you've used another programming language before, you've probably used indexes while looping. And when building new apps we will need to choose a backend to go with Angular. I'm writing something like an assembly code interpreter. What is the point of Thrower's Bandolier? How do I concatenate two lists in Python? It is used to iterate over a sequence (list, tuple, string, etc.) Long answer: No, but this does what you want: As you can see, 5 gets repeated. Or you can use list comprehensions (or map), unless you really want to mutate in place (just dont insert or remove items from the iterated-on list). How to access an index in Python for loop? So, then we need to know if what you actually want is the index and item for each item in a list, or whether you really want numbers starting from 1. How can I delete a file or folder in Python? Finally, you print index and value. Did any DOS compatibility layers exist for any UNIX-like systems before DOS started to become outmoded? How do I go about it? According to the question, one should also be able go back and forth in a loop. A for loop assigns a variable (in this case i) to the next element in the list/iterable at the start of each iteration. @TheRealChx101 according to my tests (Python 3.6.3) the difference is negligible and sometimes even in favour of, @TheRealChx101: It's lower than the overhead of looping over a. How to modify the code so that the value of the array is changed? Python is a very high-level programming language, and it tends to stray away from anything remotely resembling internal data structure. Should we edit a question to transcribe code from an image to text? What does the "yield" keyword do in Python? All you need in the for loop is a variable counting from 0 to 4 like so: Keep in mind that I wrote 0 to 5 because the loop stops one number before the maximum. enumerate() is mostly used in for loops where it is used to get the index along with the corresponding element over the given range. Meaning that 1 from the, # first list will be paired with 'A', 2 will be paired. You can get the values of that column in order by specifying a column of pandas.DataFrame and applying it to a for loop. Why are physically impossible and logically impossible concepts considered separate in terms of probability? In a for loop how to send the i few loops back upon a condition. We can achieve the same in Python with the following . Loop Through Index of pandas DataFrame in Python (Example) In this tutorial, I'll explain how to iterate over the row index of a pandas DataFrame in the Python programming language. This means that no matter what you do inside the loop, i will become the next element. Let's quickly jump onto the implementation part of it. How about updating the answer to Python 3? What does the "yield" keyword do in Python? totally agreed that it won't work for duplicate elements in the list. Now, let's take a look at the code which illustrates how this method is used: What we did in this example was enumerate every value in a list with its corresponding index, creating an enumerate object. What we did in this example was enumerate every value in a list with its corresponding index, creating an enumerate object. How do I loop through or enumerate a JavaScript object? On each increase, we access the list on that index: Here, we don't iterate through the list, like we'd usually do. It's pretty simple to start it from 1 other than 0: Here's how you can access the indices with their corresponding array's elements using for loops, while loops and some looping functions. Series.reindex () Method is used for changing the data on the basis of indexes. In my current situation the relationships between the object lengths is meaningful to my application. It handles nested loops better than the other examples. How to Transpose list of tuples in Python, How to calculate Euclidean distance of two points in Python, How to resize an image and keep its aspect ratio, How to generate a list of random integers bwtween 0 to 9 in Python. You can replace it with anything . Do comment if you have any doubts and suggestions on this Python for loop code. What can a lawyer do if the client wants him to be acquitted of everything despite serious evidence? We can do this by using the range() function. Python list indices start at 0 and go all the way to the length of the list minus 1. Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. Nope, not with what you have written here. TRY IT! Example: Yes, we can only if we dont change the reference of the object that we are using. There are 4 ways to check the index in a for loop in Python: The enumerate function is one of the most convenient and readable ways to check the index in for loop when iterating over a sequence in Python. Does a summoned creature play immediately after being summoned by a ready action? Use the python enumerate () function to access the index in for loop. Why not upload images of code/errors when asking a question? Changelog 2.3.0 What's Changed * Fix missing URL import for the Stream class example in README by hiohiohio in https . No spam ever. Trying to understand how to get this basic Fourier Series. Making statements based on opinion; back them up with references or personal experience. for i in range(df.shape[0] - 1, -1, -1): rowSeries = df.iloc[i] print(rowSeries.values) Output: ['Aadi' 16 'New York' 11] ['Riti' 31 'Delhi' 7] ['jack' 34 'Sydney' 5] Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide, Note that indexes in python start from 0, so the indexes for your example list are 0 to 4 not 1 to 5. Unlike, JavaScript, C, Java, and many other programming languages we don't have traditional C-style for loops. acknowledge that you have read and understood our, Data Structure & Algorithm Classes (Live), Data Structure & Algorithm-Self Paced(C++/JAVA), Android App Development with Kotlin(Live), Full Stack Development with React & Node JS(Live), GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, Adding new column to existing DataFrame in Pandas, How to get column names in Pandas dataframe, Python program to convert a list to string, Reading and Writing to text files in Python, Different ways to create Pandas Dataframe, isupper(), islower(), lower(), upper() in Python and their applications, Python | Program to convert String to a List, Check if element exists in list in Python, How to drop one or multiple columns in Pandas Dataframe, How to add time onto a DateTime object in Python, Predicting Stock Price Direction using Support Vector Machines. To help beginners out, don't confuse. But when we displayed the data in DataFrame but it still remains as previous because the operation performed was not saved as it is a temporary operation. Lists, a built-in type in Python, are also capable of storing multiple values. @drum if you need to do anything more complex than occasionally skipping forwards, then most likely the. Please see different approaches which can be used to iterate over list and access index value and their performance metrics (which I suppose would be useful for you) in code samples below: See performance metrics for each method below: As the result, using enumerate method is the fastest method for iteration when the index needed. Python programming language supports the differenttypes of loops, the loops can be executed indifferent ways. Here we are accessing the index through the list of elements. Now that we've explained how this function works, let's use it to solve our task: In this example, we passed a sequence of numbers in the range from 0 to len(my_list) as the first parameter of the zip() function, and my_list as its second parameter. I have been working with Python for a long time and I have expertise in working with various libraries on Tkinter, Pandas, NumPy, Turtle, Django, Matplotlib, Tensorflow, Scipy, Scikit-Learn, etc I have experience in working with various clients in countries like United States, Canada, United Kingdom, Australia, New Zealand, etc. @calculuswhiz the while loop is an important code snippet. The whilewhile loop has no such restriction. Python programming language supports the differenttypes of loops, the loops can be executed indifferent ways. Why do many companies reject expired SSL certificates as bugs in bug bounties? We can access the index in Python by using: The index element is used to represent the location of an element in a list. The loop variable, also known as the index, is used to reference the current item in the sequence. Using a for loop, iterate through the length of my_list. This will create 7 separate lists containing the index and its corresponding value in my_list that will be printed. Is it possible to create a concave light? when you change the value of number it does not change the value here: range (2,number+1) because this is an expression that has already been evaluated and has returned a list of numbers which is being looped over - Anentropic Is it plausible for constructed languages to be used to affect thought and control or mold people towards desired outcomes? You can also get the values of multiple columns with the built-in zip () function. end (Optional) - The position from where the search ends. A single execution of the algorithm will find the lengths (summed weights) of shortest . In computer science, the Floyd-Warshall algorithm (also known as Floyd's algorithm, the Roy-Warshall algorithm, the Roy-Floyd algorithm, or the WFI algorithm) is an algorithm for finding shortest paths in a directed weighted graph with positive or negative edge weights (but with no negative cycles). The index () method finds the first occurrence of the specified value. Remember to increase the index by 1 after each iteration. Continue statement will continue to print out the statement, and prints out the result as per the condition set. Fortunately, in Python, it is easy to do either or both. Use enumerate to get the index with the element as you iterate: And note that Python's indexes start at zero, so you would get 0 to 4 with the above. Your email address will not be published. Why is there a voltage on my HDMI and coaxial cables? vegan) just to try it, does this inconvenience the caterers and staff? If we didnt specify index values to the DataFrame while creation then it will take default values i.e. Using Kolmogorov complexity to measure difficulty of problems? This is also the safest option in my opinion because the chance of going into infinite recursion has been eliminated. Hi. Time complexity: O(n), where n is the number of iterations.Auxiliary space: O(1), as only a constant amount of extra space is used to store the value of i in each iteration. It returns a zip object - an iterator of tuples in which the first item in each passed iterator is paired together, the second item in each passed iterator is paired together, and analogously for the rest of them: The length of the iterator that this function returns is equal to the length of the smallest of its parameters. There's much more to know. The easiest way to fix your code is to iterate over the indexes: You will also learn about the keyword you can use while writing loops in Python. Let's take a look at this example: What we did in this example was use the list() constructor. Nowadays, the current idiom is enumerate, not the range call. Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide. The whilewhile loop has no such restriction. There is "for" loop which is similar to each loop in other languages. What does the * operator mean in a function call? Python why loop behaviour doesn't change if I change the value inside loop. How to Define an Auto Increment Primary Key in PostgreSQL using Python? We iterate from 0..len(my_list) with the index. The syntax of the for loop is: for val in sequence: # statement (s) Here, val accesses each item of sequence on each iteration. AllPython Examplesare inPython3, so Maybe its different from python 2 or upgraded versions. I would like to change the angle \k of the sections which are plotted with: Pass two loop variables index and val in the for loop. iDiTect All rights reserved. ; Three-expression for loops are popular because the expressions specified for the three parts can be nearly anything, so this has quite a bit more flexibility than the simpler numeric range form shown above. Staging Ground Beta 1 Recap, and Reviewers needed for Beta 2. Index is used to uniquely identify a row in Pandas DataFrame. The basic syntax or the formula of for loops in Python looks like this: for i in data: do something i stands for the iterator. Switch Case Statement in Python (Alternatives), Count numbers in string in Python [5 Methods]. Here we are accessing the index through the list of elements. I want to know if is it possible to change the value of the iterator in its for-loop? Simple idea is that i takes a value after every iteration irregardless of what it is assigned to inside the loop because the loop increments the iterating variable at the end of the iteration and since the value of i is declared inside the loop, it is simply overwritten. Find centralized, trusted content and collaborate around the technologies you use most. If you do decide you actually need some kind of counting as you're looping, you'll want to use the built-in enumerate function.
Unsupervised Probation Nc Drug Test,
Stockton Arsonist Frank,
Seraphiel Fallen Angel,
Islamic Thank You Quotes For Friends,
Articles H