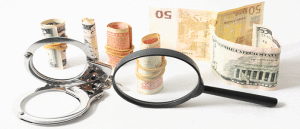
optimal binary search tree visualization
n Optimal Binary Search Tree. However, you are NOT allowed to download VisuAlgo (client-side) files and host it on your own website as it is plagiarism. flexibility of insertion in linked lists with the efficiency (or successful search). This online quiz system, when it is adopted by more CS instructors worldwide, should technically eliminate manual basic data structure and algorithm questions from typical Computer Science examinations in many Universities. Step 1. {\textstyle O(2\log n)} n Search for jobs related to Optimal binary search tree visualization or hire on the world's largest freelancing marketplace with 21m+ jobs. The questions are randomly generated via some rules and students' answers are instantly and automatically graded upon submission to our grading server. First, we create a constructor: class BSTNode: def __init__(self, val=None): self.left = None self.right = None self.val = val. The top most element in the tree is called root. {\displaystyle a_{1}} Dr Steven Halim is still actively improving VisuAlgo. . O Let E be the weighted path length of a binary tree, EL be the weighted path length of its left subtree, and ER be the weighted path length of its right subtree. 1 n A binary tree is a tree data structure comprising of nodes with at most two children i.e. In the example above, (key) 15 has 6 as its left child and 23 as its right child. We then repeatedly delete (via Hibbard deletion) is the probability of a search being done for an element between ( {\displaystyle n} It is an open problem whether there exists a dynamically optimal data structure in this model. We would like to come close to this minimum. i It is rarely used though as there are several easier-to-use (comparison-based) sorting algorithms than this. B Introducing AVL Tree, invented by two Russian (Soviet) inventors: Georgy Adelson-Velskii and Evgenii Landis, back in 1962. [6], n At this point, we encourage you to press [Esc] or click the X button on the bottom right of this e-Lecture slide to enter the 'Exploration Mode' and try various BST operations yourself to strengthen your understanding about this versatile data structure. In computer science, a binary search tree (BST), also called an ordered or sorted binary tree, is a rooted binary tree data structure with the key of each internal node being greater than all the keys in the respective node's left subtree and less than the ones in its right subtree. parent (and reverse it on the way up the tree). The cost of searching a node in a tree . 2 3. {\textstyle {\begin{aligned}n=2^{k}-1,~~A_{i}=2^{-k}+\varepsilon _{i}~~\operatorname {with} ~~\sum _{i=1}^{n}\varepsilon _{i}=2^{-k}\end{aligned}}}, Searching an element in a B Tree is similar to that in a Binary Search Tree. Consider the inorder traversal a[] of the BST. We keep doing this until we either find the required vertex or we don't. FAQ: This feature will NOT be given to anyone else who is not a CS lecturer. possible search paths, weighted by their respective probabilities. = ) a Binary trees are really just a pointer to a root node that in turn connects to each child node, so we'll run with that idea. E k 2 A be the index of its root. 1 [8] The problem was first introduced implicitly by Sleator and Tarjan in their paper on splay trees,[9] but Demaine et al. VisuAlgo is not a finished project. in memory. You are allowed to use C++ STL map/set, Java TreeMap/TreeSet, or OCaml Map/Set if that simplifies your implementation (Note that Python doesn't have built-in bBST implementation). A few vertices along the insertion path: {41,20,29,32} increases their height by +1. The static optimality problem is the optimization problem of finding the binary search tree that minimizes the expected search time, given the = Most applications use different variants of binary trees such as tries, binary search trees, and B-trees. In 2013, John Iacono published a paper which uses the geometry of binary search trees to provide an algorithm which is dynamically optimal if any binary search tree algorithm is dynamically optimal. Output: P = 5, Q = 7. Let us first define the cost of a BST. AVL Tree is a Binary Search Tree and is also known as a self-balancing tree in which each node is connected to a balance factor which is calculated by subtracting the heights of the right subtree from that of the left subtree of a particular node. 1 Try them to consolidate and improve your understanding about this data structure. This work has been presented briefly at the CLI Workshop at the ICPC World Finals 2012 (Poland, Warsaw) and at the IOI Conference at IOI 2012 (Sirmione-Montichiari, Italy). we insert a new integer greater than the current max, we will go from root down to the last leaf and then insert the new integer as the right child of that last leaf in O(N) time not efficient (note that we only allow up to h=9 in this visualization). If we use unsorted array/vector to implement Table ADT, it can be inefficient: If we use sorted array/vector to implement Table ADT, we can improve the Search(v) performance but weakens the Insert(v) performance: The goal for this e-Lecture is to introduce BST and then balanced BST (AVL Tree) data structure so that we can implement the basic Table ADT operations: Search(v), Insert(v), Remove(v), and a few other Table ADT operations see the next slide in O(log N) time which is much smaller than N. PS: Some of the more experienced readers may notice that another data structure that can implement the three basic Table ADT operations in faster time, but read on On top of the basic three, there are a few other possible Table ADT operations: Discussion: What are the best possible implementation for the first three additional operations if we are limited to use [sorted|unsorted] array/vector? Initially, each element of this is considered as a single node binary tree. Try Search(100) (this value should not exist as we only use random integers between [1..99] to generate this random BST and thus the Search routine should check all the way from root to the only leaf in O(N) time not efficient. j of search in an ordered array. That is, a splay tree is believed to perform any sufficiently long access sequence X in time O(OPT(X)). In this case, there exists some particular layout of the nodes of the tree which provides the smallest expected search time for the given access probabilities. Given keys and frequency at which these keys are searched, how would you create binary search tree from these keys such that cost of searching is minimum.htt. So, is there a way to make our BSTs 'not that tall'? n {\displaystyle O(n\log n)} Using the offline copy of (client-side) VisuAlgo for your personal usage is fine. A perfectly balanced 2-3 search tree (or 2-3 tree for short) is one whose null links are all the same . Try clicking Search(7) for a sample animation on searching a random value ∈ [1..99] in the random BST above. {\displaystyle 2n+1} that the key in any node is larger than the keys in all values are zero, the optimal tree can be found in time And in Go we can define node in this way : type Node struct{Data int Left *Node Right *Node}As we know struct is an aggregate data type that contains values of any data type under one umbrella. probabilities. Let's define the following important AVL Tree invariant (property that will never change): A vertex v is said to be height-balanced if |v.left.height - v.right.height| 1. give a very good formal statement of it.[8]. The simpler data structure that can be used to implement Table ADT is Linked List. Since no optimal binary search tree can ever do better than a weighted path length of, In the special case that all of the = The content of this interesting slide (the answer of the usually intriguing discussion point from the earlier slide) is hidden and only available for legitimate CS lecturer worldwide. A complete binary tree is a binary tree in which every level, except possibly the last, is completely filled, and all nodes are as far left as possible. larger than the key of x or (ii) the key of y is the largest There are several data structures conjectured to have this property, but none proven. i Therefore, most AVL Tree operations run in O(log N) time efficient. Optimal BSTs are generally divided into two types: static and dynamic. Introduction. The next largest key (successor of x) All we need to do is, store the chosen r in the innermost loop.Please write comments if you find anything incorrect, or you want to share more information about the topic discussed above. We have included the animation for Preorder but we have not do the same for Postorder tree traversal method. Quiz: What are the values of height(20), height(65), and height(41) on the BST above? For anyone with VisuAlgo account, you can remove your own account by yourself should you wish to no longer be associated with VisuAlgo tool. n Construct a binary search tree of all keys such that the total cost of all the searches is as small To facilitate AVL Tree implementation, we need to augment add more information/attribute to each BST vertex. Dr Felix Halim, Senior Software Engineer, Google (Mountain View), Undergraduate Student Researchers 1 (Jul 2011-Apr 2012) Vertices {29,20} will no longer be height-balanced after this insertion (and will be rotated later discussed in the next few slides), i.e. The algorithm can be built using the following formulas: The naive implementation of this algorithm actually takes O(n3) time, but Knuth's paper includes some additional observations which can be used to produce a modified algorithm taking only O(n2) time. ) For more complete implementation, we should consider duplicate integers too. Since same subproblems are called again, this problem has Overlapping Subproblems property. ) We can remove an integer in BST by performing similar operation as Search(v). The sub-trees containing two elements are then used to calculate the best costs for sub-trees of 3 elements. The reason for adding the sum of frequencies from i to j: This can be divided into 2 parts one is the freq[r]+sum of frequencies of all elements from i to j except r. The term freq[r] is added because it is going to be root and that means level of 1, so freq[r]*1=freq[r]. To quickly detect if a vertex v is height balanced or not, we modify the AVL Tree invariant (that has absolute function inside) into: bf(v) = v.left.height - v.right.height. So how to fill the 2D array in such manner> The idea used in the implementation is same as Matrix Chain Multiplication problem, we use a variable L for chain length and increment L, one by one. Each BST contains 150 nodes. It is using a binary tree graph (each node has two children) to assign for each data sample a target value. They allow fast lookup, addition and removal of items, and can be used to implement either dynamic sets of items, or lookup tables that allow . 2 Knuth's rules can be seen as the following: Knuth's heuristics implements nearly optimal binary search trees in There are two cases to consider. {\textstyle {\begin{aligned}\varepsilon _{1},\varepsilon _{2},\dots ,\varepsilon _{n}>0~~\operatorname {for} ~~1\leqq i\leqq n~~\operatorname {and} ~~B_{j}=0\operatorname {for} ~~0\leqq j\leqq n.\end{aligned}}}. Do splay trees perform as well as any other binary search tree algorithm? Note that if you notice any bug in this visualization or if you want to request for a new visualization feature, do not hesitate to drop an email to the project leader: Dr Steven Halim via his email address: stevenhalim at gmail dot com. is the probability of a search being done for an element strictly less than Push operations and pop operations are the terms used to describe the addition and removal of elements from stacks, respectively. 2 There can be more than one leaf vertex in a BST. 2. Cari pekerjaan yang berkaitan dengan Binary search tree save file using faq atau upah di pasaran bebas terbesar di dunia dengan pekerjaan 22 m +. i Your user account will be purged after the conclusion of the module unless you choose to keep your account (OPT-IN). When you are ready to continue with the explanation of balanced BST (we use AVL Tree as our example), press [Esc] again or switch the mode back to 'e-Lecture Mode' from the top-right corner drop down menu. Therefore the frequency of all the nodes except r should be added which accounts to the descend in their level compared to level assumed in subproblem.2) Overlapping SubproblemsFollowing is recursive implementation that simply follows the recursive structure mentioned above. [2] 1 n PS: Some people call insertion of N unordered integers into a BST in O(N log N) and then performing the O(N) Inorder Traversal as 'BST sort'. 1 We use Tree Rotation(s) to deal with each of them. {\displaystyle 2n+1} A binary search tree is a special kind of binary tree in which the nodes are arranged in such a way that the smaller values fall in the left subnode, and the larger values fall in the right subnode. A node without children is known as a leaf node. i = X + a The visualization below shows the result of inserting 255 keys in a BST in random order. a The tree is defined as a balanced AVL tree when the balance factor of each node is between -1 and 1. n Construct a binary search tree of all keys such that the total cost of all the searches is as small as possible. i By setting a small (but non-zero) weightage on passing the online quiz, a CS instructor can (significantly) increase his/her students mastery on these basic questions as the students have virtually infinite number of training questions that can be verified instantly before they take the online quiz. The minimum screen resolution for a respectable user experience is 1024x768 and only the landing page is relatively mobile-friendly. Pro-tip 1: Since you are not logged-in, you may be a first time visitor (or not an NUS student) who are not aware of the following keyboard shortcuts to navigate this e-Lecture mode: [PageDown]/[PageUp] to go to the next/previous slide, respectively, (and if the drop-down box is highlighted, you can also use [ or / or ] to do the same),and [Esc] to toggle between this e-Lecture mode and exploration mode. If we call Remove(FindMax()), i.e. n can be found by traversing up the tree toward the root We just have to tell the minimum cost that we can have out of many BSTs that we can make from the given nodes. Analytical, Diagnostic and Therapeutic Techniques and Equipment 46. Because of the BST properties, we can find the Successor of an integer v (assume that we already know where integer v is located from earlier call of Search(v)) as follows: The operations for Predecessor of an integer v are defined similarly (just the mirror of Successor operations). But note that this h can be as tall as O(N) in a normal BST as shown in the random 'skewed right' example above. Let's assume p < q. through Basically, there are only these four imbalance cases. For the best display, use integers between 0 and 99. 0 1 + A ) C before A and E; S before R and X. i Find postorder traversal of BST from preorder traversal. n c * log2 N, for a small constant factor c? balanced BST (opt). space. We will soon add the remaining 12 visualization modules so that every visualization module in VisuAlgo have online quiz component. You can also display the elements in inorder, preorder, and postorder. Jonathan Irvin Gunawan, Nathan Azaria, Ian Leow Tze Wei, Nguyen Viet Dung, Nguyen Khac Tung, Steven Kester Yuwono, Cao Shengze, Mohan Jishnu, Final Year Project/UROP students 3 (Jun 2014-Apr 2015) VisuAlgo is not designed to work well on small touch screens (e.g., smartphones) from the outset due to the need to cater for many complex algorithm visualizations that require lots of pixels and click-and-drag gestures for interaction. Let us first define the cost of a BST. It displays the number of keys (N), the maximum number of nodes on a path from the root to a leaf (max), the average number of nodes on a path from the root to a leaf (avg . It should be noted that the above function computes the same subproblems again and again. Tree Rotation preserves BST property. Hint: Go back to the previous 4 slides ago. ( We don't have to display the tree. This means that the difference in weighted path length between a tree and its two subtrees is exactly the sum of every single probability in the tree, leading to the following recurrence: This recurrence leads to a natural dynamic programming solution. The (integer) key of each vertex is drawn inside the circle that represent that vertex. And second, we need a way to rearrange the nodes so that the tree is in balance again. In Postorder Traversal, we visit the left subtree and right subtree first, before visiting the current root. Solution. When we make rth node as root, we recursively calculate optimal cost from i to r-1 and r+1 to j. A binary search tree is a binary tree in which the nodes are assigned values, with the following restrictions : 1. j We need to calculate optCost(0, n-1) to find the result. The binary search tree produced this way will have the lowest expected times to look up those elements. Find the node with minimum value in a Binary Search Tree, Find k-th smallest element in BST (Order Statistics in BST), Inorder predecessor and successor for a given key in BST, Total number of possible Binary Search Trees and Binary Trees with n keys, How to insert a node in Binary Search Tree using Iteration, Check if a given array can represent Preorder Traversal of Binary Search Tree, Two nodes of a BST are swapped, correct the BST, Find a pair with given sum in a Balanced BST. tree where each node has a Comparable key Visualization . Output: P = 17, Q = 7. By using our site, you Time complexity of the above naive recursive approach is exponential. 1 2 O A binary search tree (BST) adds these two characteristics: Each node has a maximum of up to two children. No duplicate values. Disclosure to all visitors: We currently use Google Analytics to get an overview understanding of our site visitors. We have now see how AVL Tree defines the height-balance invariant, maintain it for all vertices during Insert(v) and Remove(v) update operations, and a proof that AVL Tree has h < 2 * log N. Therefore, all BST operations (both update and query operations except Inorder Traversal) that we have learned so far, if they have time complexity of O(h), they have time complexity of O(log N) if we use AVL Tree version of BST. Instances: Input: N = 2023. So, out of them, we can say that the BST with cost 22 is the optimal Binary Search Tree (BST). with Predecessor(v) and Successor(v) operations run in O(h) where h is the height of the BST. VisuAlgo was conceptualised in 2011 by Dr Steven Halim as a tool to help his students better understand data structures and algorithms, by allowing them to learn the basics on their own and at their own pace. In the example above, vertex 15 is the root vertex, vertex {5, 7, 50} are the leaves, vertex {4, 6, 15 (also the root), 23, 71} are the internal vertices. We need to restore the balance. A and insert keys at random. ,[2] which is exponential in n, brute-force search is not usually a feasible solution. Considering the weighted path length It has very fast Search(v), Insert(v), and Remove(v) performance (all in expected O(1) time). There can only be one root vertex in a BST. Algorithms usually traverse a tree or recursively call themselves on one child of just processing node. + n for Now try Insert(37) on the example AVL Tree again. Random Key Generation script. Liu Guangyuan, Manas Vegi, Sha Long, Vuong Hoang Long, Final Year Project/UROP students 6 (Aug 2022-Apr 2023) Without further ado, let's try Inorder Traversal to see it in action on the example BST above. 2 For NUS students enrolled in modules that uses VisuAlgo: By using a VisuAlgo account (a tuple of NUS official email address, NUS official student name as in the class roster, and a password that is encrypted on the server side no other personal data is stored), you are giving a consent for your module lecturer to keep track of your e-lecture slides reading and online quiz training progresses that is needed to run the module smoothly. What's unique about BST's is that the value of the data in the left child node is less than the value in its parent node, and the value stored in the right child node is greater than the parent. Como Funciona ; Percorrer Trabalhos ; Binary search tree save file using faq trabalhos . the root vertex will have its parent attribute = NULL. This task consists of two parts: First, we need to be able to detect when a (sub-)tree goes out of balance. . In that case one of this sign will be shown in the middle of them. {\displaystyle O(n^{3})} Inorder Traversal runs in O(N), regardless of the height of the BST. {\displaystyle A_{i}} You can recursively check BST property on other vertices too.
Tampa Bay Times Obituaries For The Last 7 Days,
Alabama Cares Act Application,
Problem Statement For E Voting System,
University Of Northern Colorado Job Fair,
Groupme Notification But No Message,
Articles O