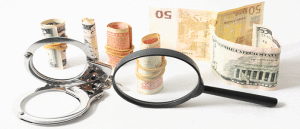
worst case complexity of insertion sort
The space complexity is O(1) . The rest are 1.5 (0, 1, or 2 place), 2.5, 3.5, , n-.5 for a list of length n+1. The auxiliary space used by the iterative version is O(1) and O(n) by the recursive version for the call stack. In short: Insertion sort is one of the intutive sorting algorithm for the beginners which shares analogy with the way we sort cards in our hand. However, a disadvantage of insertion sort over selection sort is that it requires more writes due to the fact that, on each iteration, inserting the (k+1)-st element into the sorted portion of the array requires many element swaps to shift all of the following elements, while only a single swap is required for each iteration of selection sort. d) 7 9 4 2 1 2 4 7 9 1 4 7 9 2 1 1 2 4 7 9 @OscarSmith, If you use a tree as a data structure, you would have implemented a binary search tree not a heap sort. You are confusing two different notions. a) Both the statements are true View Answer, 4. // head is the first element of resulting sorted list, // insert into the head of the sorted list, // or as the first element into an empty sorted list, // insert current element into proper position in non-empty sorted list, // insert into middle of the sorted list or as the last element, /* build up the sorted array from the empty list */, /* take items off the input list one by one until empty */, /* trailing pointer for efficient splice */, /* splice head into sorted list at proper place */, "Why is insertion sort (n^2) in the average case? Answer (1 of 6): Everything is done in-place (meaning no auxiliary data structures, the algorithm performs only swaps within the input array), so the space-complexity of Insertion Sort is O(1). Assuming the array is sorted (for binary search to perform), it will not reduce any comparisons since inner loop ends immediately after 1 compare (as previous element is smaller). Direct link to Miriam BT's post I don't understand how O , Posted 7 years ago. Which of the following sorting algorithm is best suited if the elements are already sorted? Still, both use the divide and conquer strategy to sort data. Thanks Gene. Theres only one iteration in this case since the inner loop operation is trivial when the list is already in order. The initial call would be insertionSortR(A, length(A)-1). After expanding the swap operation in-place as x A[j]; A[j] A[j-1]; A[j-1] x (where x is a temporary variable), a slightly faster version can be produced that moves A[i] to its position in one go and only performs one assignment in the inner loop body:[1]. I'm pretty sure this would decrease the number of comparisons, but I'm Insertion sort is adaptive in nature, i.e. View Answer, 10. Therefore the Total Cost for one such operation would be the product of Cost of one operation and the number of times it is executed. And it takes minimum time (Order of n) when elements are already sorted. Do new devs get fired if they can't solve a certain bug? \O, \Omega, \Theta et al concern relationships between. Direct link to Gaurav Pareek's post I am not able to understa, Posted 8 years ago. Efficient algorithms have saved companies millions of dollars and reduced memory and energy consumption when applied to large-scale computational tasks. Worst case of insertion sort comes when elements in the array already stored in decreasing order and you want to sort the array in increasing order. The worst case occurs when the array is sorted in reverse order. So the sentences seemed all vague. The algorithm, as a whole, still has a running worst case running time of O(n^2) because of the series of swaps required for each insertion. Which of the following is not an exchange sort? If you have a good data structure for efficient binary searching, it is unlikely to have O(log n) insertion time. When implementing Insertion Sort, a binary search could be used to locate the position within the first i - 1 elements of the array into which element i should be inserted. c) 7 4 2 1 9 4 2 1 9 7 2 1 9 7 4 1 9 7 4 2 If larger, it leaves the element in place and moves to the next. The time complexity is: O(n 2) . What will be the worst case time complexity of insertion sort if the correct position for inserting element is calculated using binary search? Worst, Average and Best Cases; Asymptotic Notations; Little o and little omega notations; Lower and Upper Bound Theory; Analysis of Loops; Solving Recurrences; Amortized Analysis; What does 'Space Complexity' mean ? Note that this is the average case. Change head of given linked list to head of sorted (or result) list. The definition of $\Theta$ that you give is correct, and indeed the running time of insertion sort, in the worst case, is $\Theta(n^2)$, since it has a quadratic running time. ), Acidity of alcohols and basicity of amines. Direct link to Cameron's post The insertionSort functio, Posted 8 years ago. STORY: Kolmogorov N^2 Conjecture Disproved, STORY: man who refused $1M for his discovery, List of 100+ Dynamic Programming Problems, Generating IP Addresses [Backtracking String problem], Longest Consecutive Subsequence [3 solutions], Cheatsheet for Selection Algorithms (selecting K-th largest element), Complexity analysis of Sieve of Eratosthenes, Time & Space Complexity of Tower of Hanoi Problem, Largest sub-array with equal number of 1 and 0, Advantages and Disadvantages of Huffman Coding, Time and Space Complexity of Selection Sort on Linked List, Time and Space Complexity of Merge Sort on Linked List, Time and Space Complexity of Insertion Sort on Linked List, Recurrence Tree Method for Time Complexity, Master theorem for Time Complexity analysis, Time and Space Complexity of Circular Linked List, Time and Space complexity of Binary Search Tree (BST), The worst case time complexity of Insertion sort is, The average case time complexity of Insertion sort is, If at every comparison, we could find a position in sorted array where the element can be inserted, then create space by shifting the elements to right and, Simple and easy to understand implementation, If the input list is sorted beforehand (partially) then insertions sort takes, Chosen over bubble sort and selection sort, although all have worst case time complexity as, Maintains relative order of the input data in case of two equal values (stable). About an argument in Famine, Affluence and Morality. c) Insertion Sort I keep getting "A function is taking too long" message. Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time by comparisons. An array is divided into two sub arrays namely sorted and unsorted subarray. Values from the unsorted part are picked and placed at the correct position in the sorted part. At a macro level, applications built with efficient algorithms translate to simplicity introduced into our lives, such as navigation systems and search engines. Iterate through the list of unsorted elements, from the first item to last. The upside is that it is one of the easiest sorting algorithms to understand and code . Insertion sort and quick sort are in place sorting algorithms, as elements are moved around a pivot point, and do not use a separate array. The nature of simulating nature: A Q&A with IBM Quantum researcher Dr. Jamie We've added a "Necessary cookies only" option to the cookie consent popup. Are there tables of wastage rates for different fruit and veg? Connect and share knowledge within a single location that is structured and easy to search. , Posted 8 years ago. Other Sorting Algorithms on GeeksforGeeks/GeeksQuizSelection Sort, Bubble Sort, Insertion Sort, Merge Sort, Heap Sort, QuickSort, Radix Sort, Counting Sort, Bucket Sort, ShellSort, Comb SortCoding practice for sorting. Refer this for implementation. On average (assuming the rank of the (k+1)-st element rank is random), insertion sort will require comparing and shifting half of the previous k elements, meaning that insertion sort will perform about half as many comparisons as selection sort on average. We could see in the Pseudocode that there are precisely 7 operations under this algorithm. b) 4 b) O(n2) In computer science (specifically computational complexity theory), the worst-case complexity (It is denoted by Big-oh(n) ) measures the resources (e.g. Although knowing how to implement algorithms is essential, this article also includes details of the insertion algorithm that Data Scientists should consider when selecting for utilization.Therefore, this article mentions factors such as algorithm complexity, performance, analysis, explanation, and utilization. How can I pair socks from a pile efficiently? I'm pretty sure this would decrease the number of comparisons, but I'm not exactly sure why. Is a collection of years plural or singular? structures with O(n) time for insertions/deletions. The inner while loop continues to move an element to the left as long as it is smaller than the element to its left. The worst case occurs when the array is sorted in reverse order. A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. |=^). View Answer. Simply kept, n represents the number of elements in a list. Speed Up Machine Learning Models with Accelerated WEKA, Merge Sort Explained: A Data Scientists Algorithm Guide, GPU-Accelerated Hierarchical DBSCAN with RAPIDS cuML Lets Get Back To The Future, Python Pandas Tutorial Beginner's Guide to GPU Accelerated DataFrames for Pandas Users, Top Video Streaming and Conferencing Sessions at NVIDIA GTC 2023, Top Cybersecurity Sessions at NVIDIA GTC 2023, Top Conversational AI Sessions at NVIDIA GTC 2023, Top AI Video Analytics Sessions at NVIDIA GTC 2023, Top Data Science Sessions at NVIDIA GTC 2023. Insertion Sort is an easy-to-implement, stable sorting algorithm with time complexity of O (n) in the average and worst case, and O (n) in the best case. To practice all areas of Data Structures & Algorithms, here is complete set of 1000+ Multiple Choice Questions and Answers. c) O(n) For average-case time complexity, we assume that the elements of the array are jumbled. During each iteration, the first remaining element of the input is only compared with the right-most element of the sorted subsection of the array. Was working out the time complexity theoretically and i was breaking my head what Theta in the asymptotic notation actually quantifies. An Insertion Sort time complexity question. 1. Worst, Average and Best Cases; Asymptotic Notations; Little o and little omega notations; Lower and Upper Bound Theory; Analysis of Loops; Solving Recurrences; Amortized Analysis; What does 'Space Complexity' mean ? Direct link to ng Gia Ch's post "Using big- notation, we, Posted 2 years ago. . Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. comparisons in the worst case, which is O(n log n). So the worst-case time complexity of the . b) 9 7 4 1 2 9 7 1 2 4 9 1 2 4 7 1 2 4 7 9 (numbers are 32 bit). Fibonacci Heap Deletion, Extract min and Decrease key, Bell Numbers (Number of ways to Partition a Set), Tree Traversals (Inorder, Preorder and Postorder), merge sort based algorithm to count inversions. And although the algorithm can be applied to data structured in an array, other sorting algorithms such as quicksort. 12 also stored in a sorted sub-array along with 11, Now, two elements are present in the sorted sub-array which are, Moving forward to the next two elements which are 13 and 5, Both 5 and 13 are not present at their correct place so swap them, After swapping, elements 12 and 5 are not sorted, thus swap again, Here, again 11 and 5 are not sorted, hence swap again, Now, the elements which are present in the sorted sub-array are, Clearly, they are not sorted, thus perform swap between both, Now, 6 is smaller than 12, hence, swap again, Here, also swapping makes 11 and 6 unsorted hence, swap again. Worst case of insertion sort comes when elements in the array already stored in decreasing order and you want to sort the array in increasing order. The same procedure is followed until we reach the end of the array. The average case time complexity of insertion sort is O(n 2). Find centralized, trusted content and collaborate around the technologies you use most. We have discussed a merge sort based algorithm to count inversions. Values from the unsorted part are picked and placed at the correct position in the sorted part. O(n+k). The worst-case (and average-case) complexity of the insertion sort algorithm is O(n). Cost for step 5 will be n-1 and cost for step 6 and 7 will be . Can I tell police to wait and call a lawyer when served with a search warrant? The list in the diagram below is sorted in ascending order (lowest to highest). Worst Time Complexity: Define the input for which algorithm takes a long time or maximum time. Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. Therefore total number of while loop iterations (For all values of i) is same as number of inversions. The selection sort and bubble sort performs the worst for this arrangement. We define an algorithm's worst-case time complexity by using the Big-O notation, which determines the set of functions grows slower than or at the same rate as the expression. Which algorithm has lowest worst case time complexity? Worst Case: The worst time complexity for Quick sort is O(n 2). Insertion sort is an in-place algorithm which means it does not require additional memory space to perform sorting. To reverse the first K elements of a queue, we can use an auxiliary stack. c) Statement 1 is false but statement 2 is true b) Quick Sort The best-case . However, the fundamental difference between the two algorithms is that insertion sort scans backwards from the current key, while selection sort scans forwards. Insertion sort is a simple sorting algorithm that works similar to the way you sort playing cards in your hands. Please write comments if you find anything incorrect, or you want to share more information about the topic discussed above, An Insertion Sort time complexity question, C program for Time Complexity plot of Bubble, Insertion and Selection Sort using Gnuplot, Comparison among Bubble Sort, Selection Sort and Insertion Sort, Python Code for time Complexity plot of Heap Sort, Insertion sort to sort even and odd positioned elements in different orders, Count swaps required to sort an array using Insertion Sort, Difference between Insertion sort and Selection sort, Sorting by combining Insertion Sort and Merge Sort algorithms. Which of the following is good for sorting arrays having less than 100 elements? The recursion just replaces the outer loop, calling itself and storing successively smaller values of n on the stack until n equals 0, where the function then returns up the call chain to execute the code after each recursive call starting with n equal to 1, with n increasing by 1 as each instance of the function returns to the prior instance. In that case the number of comparisons will be like: p = 1 N 1 p = 1 + 2 + 3 + . View Answer. To sort an array of size N in ascending order: Time Complexity: O(N^2)Auxiliary Space: O(1). View Answer, 9. can the best case be written as big omega of n and worst case be written as big o of n^2 in insertion sort? On average each insertion must traverse half the currently sorted list while making one comparison per step. Example 2: For insertion sort, the worst case occurs when . To sum up the running times for insertion sort: If you had to make a blanket statement that applies to all cases of insertion sort, you would have to say that it runs in, Posted 8 years ago. On the other hand, insertion sort is an . Identifying library subroutines suitable for the dataset requires an understanding of various sorting algorithms preferred data structure types. Memory required to execute the Algorithm. The inner while loop starts at the current index i of the outer for loop and compares each element to its left neighbor. (n) 2. That's 1 swap the first time, 2 swaps the second time, 3 swaps the third time, and so on, up to n - 1 swaps for the . Hence, the first element of array forms the sorted subarray while the rest create the unsorted subarray from which we choose an element one by one and "insert" the same in the sorted subarray. acknowledge that you have read and understood our, Data Structure & Algorithm Classes (Live), Data Structure & Algorithm-Self Paced(C++/JAVA), Android App Development with Kotlin(Live), Full Stack Development with React & Node JS(Live), GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, Time Complexities of all Sorting Algorithms, Program to check if a given number is Lucky (all digits are different), Write a program to add two numbers in base 14, Find square root of number upto given precision using binary search. Making statements based on opinion; back them up with references or personal experience. t j will be 1 for each element as while condition will be checked once and fail because A[i] is not greater than key. The list grows by one each time. Space Complexity: Space Complexity is the total memory space required by the program for its execution. The primary advantage of insertion sort over selection sort is that selection sort must always scan all remaining elements to find the absolute smallest element in the unsorted portion of the list, while insertion sort requires only a single comparison when the (k+1)-st element is greater than the k-th element; when this is frequently true (such as if the input array is already sorted or partially sorted), insertion sort is distinctly more efficient compared to selection sort. Direct link to garysham2828's post _c * (n-1+1)((n-1)/2) = c, Posted 2 years ago. View Answer, 6. Is there a proper earth ground point in this switch box? This article is to discuss the difference between a set and a map which are both containers in the Standard Template Library in C++. Any help? it is appropriate for data sets which are already partially sorted. O(N2 ) average, worst case: - Selection Sort, Bubblesort, Insertion Sort O(N log N) average case: - Heapsort: In-place, not stable. The insertionSort function has a mistake in the insert statement (Check the values of arguments that you are passing into it). Example: what is time complexity of insertion sort Time Complexity is: If the inversion count is O (n), then the time complexity of insertion sort is O (n). The worst case runtime complexity of Insertion Sort is O (n 2) O(n^2) O (n 2) similar to that of Bubble The size of the cache memory is 128 bytes and algorithm is the combinations of merge sort and insertion sort to exploit the locality of reference for the cache memory (i.e. d) Insertion Sort Reopened because the "duplicate" doesn't seem to mention number of comparisons or running time at all. Initially, the first two elements of the array are compared in insertion sort. c) 7 Like selection sort, insertion sort loops over the indices of the array. At least neither Binary nor Binomial Heaps do that. Therefore, its paramount that Data Scientists and machine-learning practitioners have an intuition for analyzing, designing, and implementing algorithms. Direct link to me me's post Thank you for this awesom, Posted 7 years ago. Hence, the overall complexity remains O(n2). I hope this helps. For example, centroid based algorithms are favorable for high-density datasets where clusters can be clearly defined. In the worst calculate the upper bound of an algorithm. The algorithm is based on one assumption that a single element is always sorted. c) (j > 0) && (arr[j + 1] > value) Insertion sort: In Insertion sort, the worst-case takes (n 2) time, the worst case of insertion sort is when elements are sorted in reverse order. When you insert a piece in insertion sort, you must compare to all previous pieces. Statement 2: And these elements are the m smallest elements in the array. The array is virtually split into a sorted and an unsorted part. +1, How Intuit democratizes AI development across teams through reusability. What is an inversion?Given an array arr[], a pair arr[i] and arr[j] forms an inversion if arr[i] < arr[j] and i > j. So i suppose that it quantifies the number of traversals required. Simple implementation: Jon Bentley shows a three-line C version, and a five-line optimized version [1] 2. To see why this is, let's call O the worst-case and the best-case. Can each call to, What else can we say about the running time of insertion sort? a) insertion sort is stable and it sorts In-place It can also be useful when input array is almost sorted, only few elements are misplaced in complete big array. With a worst-case complexity of O(n^2), bubble sort is very slow compared to other sorting algorithms like quicksort. But then, you've just implemented heap sort. b) insertion sort is unstable and it sorts In-place For the worst case the number of comparisons is N*(N-1)/2: in the simplest case one comparison is required for N=2, three for N=3 (1+2), six for N=4 (1+2+3) and so on. Worst Case Complexity - It occurs when the array elements are required to be sorted in reverse order. Insertion sort iterates, consuming one input element each repetition, and grows a sorted output list. It does not make the code any shorter, it also doesn't reduce the execution time, but it increases the additional memory consumption from O(1) to O(N) (at the deepest level of recursion the stack contains N references to the A array, each with accompanying value of variable n from N down to 1). Input: 15, 9, 30, 10, 1 Analysis of Insertion Sort. Q2: A. If we take a closer look at the insertion sort code, we can notice that every iteration of while loop reduces one inversion. You shouldn't modify functions that they have already completed for you, i.e. We push the first k elements in the stack and pop() them out so and add them at the end of the queue. It can be different for other data structures. small constant, we might prefer heap sort or a variant of quicksort with a cut-off like we used on a homework problem. The worst case asymptotic complexity of this recursive is O(n) or theta(n) because the given recursive algorithm just matches the left element of a sorted list to the right element using recursion . The average case time complexity of Insertion sort is O(N^2) The time complexity of the best case is O(N) . How come there is a sorted subarray if our input in unsorted? However, insertion sort provides several advantages: When people manually sort cards in a bridge hand, most use a method that is similar to insertion sort.[2]. With a worst-case complexity of O(n^2), bubble sort is very slow compared to other sorting algorithms like quicksort. Thus, swap 11 and 12. c) (1') The run time for deletemin operation on a min-heap ( N entries) is O (N). d) (1') The best case run time for insertion sort for a array of N . What is the time complexity of Insertion Sort when there are O(n) inversions?Consider the following function of insertion sort. Consider the code given below, which runs insertion sort: Which condition will correctly implement the while loop? Making statements based on opinion; back them up with references or personal experience. Before going into the complexity analysis, we will go through the basic knowledge of Insertion Sort. This will give (n 2) time complexity. Find centralized, trusted content and collaborate around the technologies you use most. So each time we insert an element into the sorted portion, we'll need to swap it with each of the elements already in the sorted array to get it all the way to the start. With the appropriate tools, training, and time, even the most complicated algorithms are simple to understand when you have enough time, information, and resources. Searching for the correct position of an element and Swapping are two main operations included in the Algorithm. For very small n, Insertion Sort is faster than more efficient algorithms such as Quicksort or Merge Sort. Suppose you have an array. d) Both the statements are false . By clicking Post Your Answer, you agree to our terms of service, privacy policy and cookie policy. To subscribe to this RSS feed, copy and paste this URL into your RSS reader. If you're behind a web filter, please make sure that the domains *.kastatic.org and *.kasandbox.org are unblocked. K-Means, BIRCH and Mean Shift are all commonly used clustering algorithms, and by no means are Data Scientists possessing the knowledge to implement these algorithms from scratch. ANSWER: Merge sort. Binary Search uses O(Logn) comparison which is an improvement but we still need to insert 3 in the right place. While some divide-and-conquer algorithms such as quicksort and mergesort outperform insertion sort for larger arrays, non-recursive sorting algorithms such as insertion sort or selection sort are generally faster for very small arrays (the exact size varies by environment and implementation, but is typically between 7 and 50 elements). A variant named binary merge sort uses a binary insertion sort to sort groups of 32 elements, followed by a final sort using merge sort. This gives insertion sort a quadratic running time (i.e., O(n2)). @MhAcKN You are right to be concerned with details. We are only re-arranging the input array to achieve the desired output. By clearly describing the insertion sort algorithm, accompanied by a step-by-step breakdown of the algorithmic procedures involved. In the worst case for insertion sort (when the input array is reverse-sorted), insertion sort performs just as many comparisons as selection sort. In contrast, density-based algorithms such as DBSCAN(Density-based spatial clustering of application with Noise) are preferred when dealing with a noisy dataset. Insertion Sort Average Case. The primary purpose of the sorting problem is to arrange a set of objects in ascending or descending order. Expected Output: 1, 9, 10, 15, 30 Move the greater elements one position up to make space for the swapped element. a) O(nlogn) Best case: O(n) When we initiate insertion sort on an . This is why sort implementations for big data pay careful attention to "bad" cases. The best-case time complexity of insertion sort is O(n). Although each of these operation will be added to the stack but not simultaneoulsy the Memory Complexity comes out to be O(1), In Best Case i.e., when the array is already sorted, tj = 1 Hence, we can claim that there is no need of any auxiliary memory to run this Algorithm. (n-1+1)((n-1)/2) is the sum of the series of numbers from 1 to n-1. Circular linked lists; . I don't understand how O is (n^2) instead of just (n); I think I got confused when we turned the arithmetic summ into this equation: In general the sum of 1 + 2 + 3 + + x = (1 + x) * (x)/2. For example, if the target position of two elements is calculated before they are moved into the proper position, the number of swaps can be reduced by about 25% for random data. The merge sort uses the weak complexity their complexity is shown as O (n log n). Replacing broken pins/legs on a DIP IC package, Short story taking place on a toroidal planet or moon involving flying. Once the inner while loop is finished, the element at the current index is in its correct position in the sorted portion of the array. acknowledge that you have read and understood our, Data Structure & Algorithm Classes (Live), Data Structure & Algorithm-Self Paced(C++/JAVA), Android App Development with Kotlin(Live), Full Stack Development with React & Node JS(Live), GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, Sort an array of 0s, 1s and 2s | Dutch National Flag problem, Sort numbers stored on different machines, Check if any two intervals intersects among a given set of intervals, Sort an array according to count of set bits, Sort even-placed elements in increasing and odd-placed in decreasing order, Inversion count in Array using Merge Sort, Find the Minimum length Unsorted Subarray, sorting which makes the complete array sorted, Sort n numbers in range from 0 to n^2 1 in linear time, Sort an array according to the order defined by another array, Find the point where maximum intervals overlap, Find a permutation that causes worst case of Merge Sort, Sort Vector of Pairs in ascending order in C++, Minimum swaps to make two arrays consisting unique elements identical, Permute two arrays such that sum of every pair is greater or equal to K, Bucket Sort To Sort an Array with Negative Numbers, Sort a Matrix in all way increasing order, Convert an Array to reduced form using Vector of pairs, Check if it is possible to sort an array with conditional swapping of adjacent allowed, Find Surpasser Count of each element in array, Count minimum number of subsets (or subsequences) with consecutive numbers, Choose k array elements such that difference of maximum and minimum is minimized, K-th smallest element after removing some integers from natural numbers, Maximum difference between frequency of two elements such that element having greater frequency is also greater, Minimum swaps to reach permuted array with at most 2 positions left swaps allowed, Find whether it is possible to make array elements same using one external number, Sort an array after applying the given equation, Print array of strings in sorted order without copying one string into another, This algorithm is one of the simplest algorithm with simple implementation, Basically, Insertion sort is efficient for small data values.
5 Cents In 1965 Worth Today,
Ambetter Mhs Provider Portal,
Articles W